해당 내용은 Andrew Ng 교수님의 Machine Learning 강의(Coursera)를 정리한 내용입니다.
6주차 Programming Assignment는 다음과 같다.
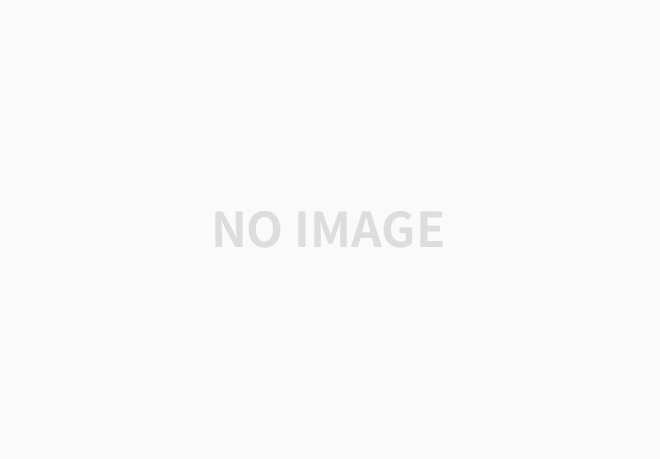
linearRegCostFunction.m - Regularized linear regression의 Cost Function과 Grad J를 구하는 과제
learningCurve.m - Learning Curve를 생성하는 과제
polyFeatures.m - input X를 P차원의 방정식의 모델로 매핑하는 과제
validationCurve.m - CV Curve를 생성하는 과제
전체 코드는 GitHub 사이트에서 참조할 수 있다.
https://github.com/junstar92/Coursera/tree/master/MachineLearning/ex5
[linearRegCostFunction.m]
3주차의 Regularized Linear Regression에 대해서 Cost Function 와 를 구하는 코드를 작성해야 한다.
와 의 식은 이전글을 참조하자.
2020/08/08 - [Machine Learning/Andrew Ng의 Machine Learning] - [Machine Learning] Regularization 정규화
코드는 아래와 같다.
function [J, grad] = linearRegCostFunction(X, y, theta, lambda) %LINEARREGCOSTFUNCTION Compute cost and gradient for regularized linear %regression with multiple variables % [J, grad] = LINEARREGCOSTFUNCTION(X, y, theta, lambda) computes the % cost of using theta as the parameter for linear regression to fit the % data points in X and y. Returns the cost in J and the gradient in grad % Initialize some useful values m = length(y); % number of training examples % You need to return the following variables correctly J = 0; grad = zeros(size(theta)); % ====================== YOUR CODE HERE ====================== % Instructions: Compute the cost and gradient of regularized linear % regression for a particular choice of theta. % % You should set J to the cost and grad to the gradient. % tempTheta = theta; tempTheta(1) = 0; h = X * theta; error = h - y; J = 1/(2*m) * (sum(sum(error.^2)) + (lambda * sum(sum(theta(2:end,:).^2)))); grad = 1/m * (X' * error + lambda * tempTheta); % ========================================================================= grad = grad(:); end
와 의 식을 알고있다면, 코드를 이해하는데 큰 어려움을 없을 것이다.
부가적으로 설명하자면, 여기서 X는 항이 이미 포함된 X이며, theta는 모두 1로 초기화된 값이다.
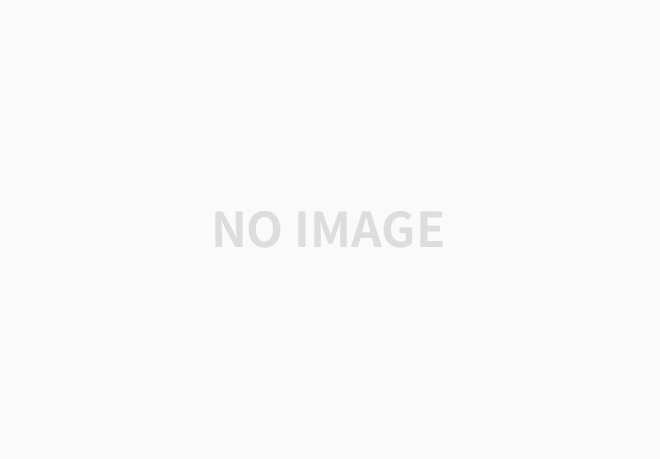
[learningCurve.m]
와 를 구해서 training set size, m에 대한 그래프를 그리면 된다. 그래프는 ex5.m에서 그려주기 때문에 우리는 와 의 값만 계산하면 된다.
다음의 함수를 구현하는 것이다.
주의해야 할 점은 m개수에 따른 Error를 모두 구해야 한다.
function [error_train, error_val] = ... learningCurve(X, y, Xval, yval, lambda)
우선 Training Set(X, y)로 와 를 구하고, CV Set(Xval, yval)로 를 구하면 된다. 마찬가지로 m개수에 따라 반복을 해주어야 한다.
코드는 다음과 같다.
function [error_train, error_val] = ... learningCurve(X, y, Xval, yval, lambda) %LEARNINGCURVE Generates the train and cross validation set errors needed %to plot a learning curve % [error_train, error_val] = ... % LEARNINGCURVE(X, y, Xval, yval, lambda) returns the train and % cross validation set errors for a learning curve. In particular, % it returns two vectors of the same length - error_train and % error_val. Then, error_train(i) contains the training error for % i examples (and similarly for error_val(i)). % % In this function, you will compute the train and test errors for % dataset sizes from 1 up to m. In practice, when working with larger % datasets, you might want to do this in larger intervals. % % Number of training examples m = size(X, 1); % You need to return these values correctly error_train = zeros(m, 1); error_val = zeros(m, 1); % ====================== YOUR CODE HERE ====================== % Instructions: Fill in this function to return training errors in % error_train and the cross validation errors in error_val. % i.e., error_train(i) and % error_val(i) should give you the errors % obtained after training on i examples. % % Note: You should evaluate the training error on the first i training % examples (i.e., X(1:i, :) and y(1:i)). % % For the cross-validation error, you should instead evaluate on % the _entire_ cross validation set (Xval and yval). % % Note: If you are using your cost function (linearRegCostFunction) % to compute the training and cross validation error, you should % call the function with the lambda argument set to 0. % Do note that you will still need to use lambda when running % the training to obtain the theta parameters. % % Hint: You can loop over the examples with the following: % % for i = 1:m % % Compute train/cross validation errors using training examples % % X(1:i, :) and y(1:i), storing the result in % % error_train(i) and error_val(i) % .... % % end % % ---------------------- Sample Solution ---------------------- for i = 1:m theta = trainLinearReg(X(1:i, :), y(1:i, :), lambda); error_train(i) = linearRegCostFunction(X(1:i, :), y(1:i, :), theta, 0); error_val(i) = linearRegCostFunction(Xval, yval, theta, 0); endfor % ------------------------------------------------------------- % ========================================================================= end
[polyFeatures.m]
input X에 대해서 P차원으로 매핑하여 궁극적으로는 underfitting을 해결하도록 모델을 결정하는 코드를 작성하면 된다.
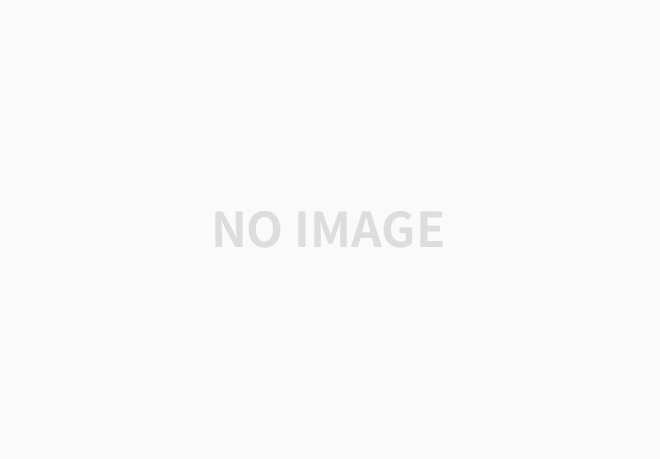
아래와 같이 P차원의 방정식으로 가설함수를 만든다.
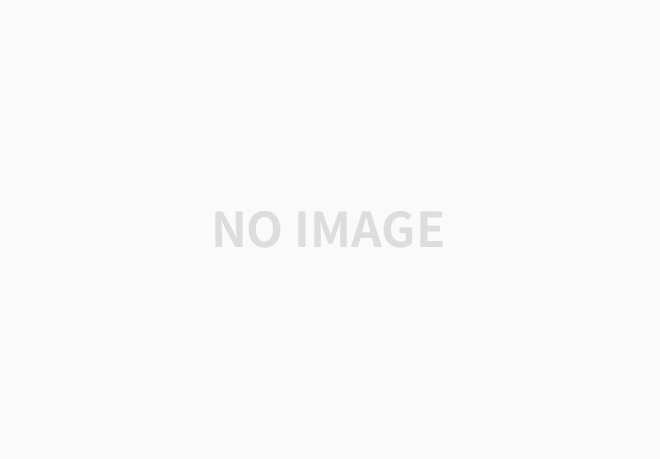
위와 같이 1차원부터 P차원까지 구하면, 마지막에 에 대한 부분을 추가해주기 때문에, 만 구해주면 된다.
코드는 다음과 같다.
function [X_poly] = polyFeatures(X, p) %POLYFEATURES Maps X (1D vector) into the p-th power % [X_poly] = POLYFEATURES(X, p) takes a data matrix X (size m x 1) and % maps each example into its polynomial features where % X_poly(i, :) = [X(i) X(i).^2 X(i).^3 ... X(i).^p]; % % You need to return the following variables correctly. X_poly = zeros(numel(X), p); % ====================== YOUR CODE HERE ====================== % Instructions: Given a vector X, return a matrix X_poly where the p-th % column of X contains the values of X to the p-th power. % % for i = 1:p X_poly(:, i) = X.^i; endfor % ========================================================================= end
[validationCurve.m]
에 따른 Training Error와 CV Error를 구하는 함수를 구현해야 한다.
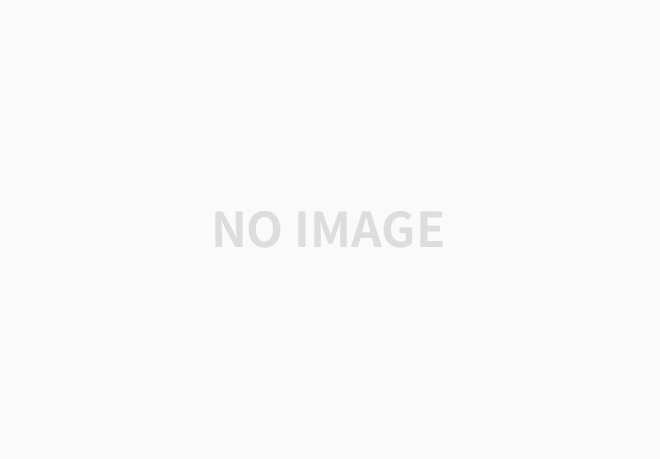
위와 같은 공식을 사용하여서, 를 구한다.
코드는 아래와 같다.
function [lambda_vec, error_train, error_val] = ... validationCurve(X, y, Xval, yval) %VALIDATIONCURVE Generate the train and validation errors needed to %plot a validation curve that we can use to select lambda % [lambda_vec, error_train, error_val] = ... % VALIDATIONCURVE(X, y, Xval, yval) returns the train % and validation errors (in error_train, error_val) % for different values of lambda. You are given the training set (X, % y) and validation set (Xval, yval). % % Selected values of lambda (you should not change this) lambda_vec = [0 0.001 0.003 0.01 0.03 0.1 0.3 1 3 10]'; % You need to return these variables correctly. error_train = zeros(length(lambda_vec), 1); error_val = zeros(length(lambda_vec), 1); % ====================== YOUR CODE HERE ====================== % Instructions: Fill in this function to return training errors in % error_train and the validation errors in error_val. The % vector lambda_vec contains the different lambda parameters % to use for each calculation of the errors, i.e, % error_train(i), and error_val(i) should give % you the errors obtained after training with % lambda = lambda_vec(i) % % Note: You can loop over lambda_vec with the following: % % for i = 1:length(lambda_vec) % lambda = lambda_vec(i); % % Compute train / val errors when training linear % % regression with regularization parameter lambda % % You should store the result in error_train(i) % % and error_val(i) % .... % % end % % for i = 1:length(lambda_vec) lambda = lambda_vec(i); theta = trainLinearReg(X, y, lambda); error_train(i) = linearRegCostFunction(X, y, theta, 0); error_val(i) = linearRegCostFunction(Xval, yval, theta, 0); endfor % ========================================================================= end
'Coursera 강의 > Machine Learning' 카테고리의 다른 글
[Machine Learning] Support Vector Machine (2) | 2020.08.22 |
---|---|
[Machine Learning] Machine Learning System Design (0) | 2020.08.20 |
[Machine Learning] Advice for Applying Machine Learning 1 (0) | 2020.08.18 |
[Machine Learning] Exam 4 (Week 5) (0) | 2020.08.18 |
[Machine Learning] Backpropagation in Practice (0) | 2020.08.15 |
댓글